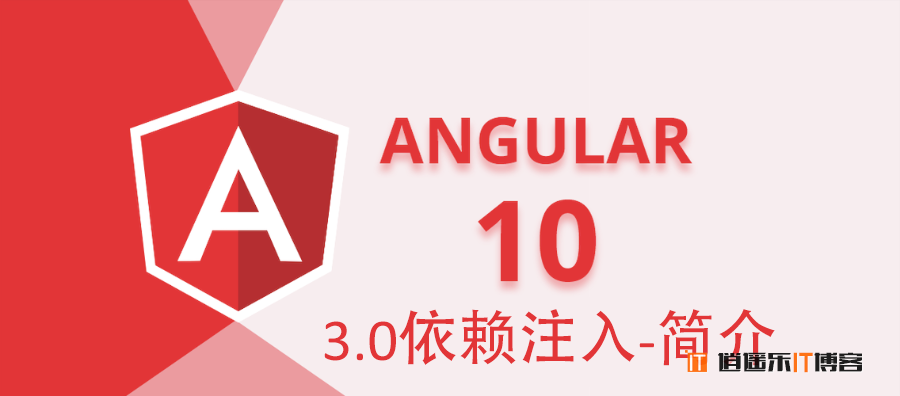
题外话-带修饰符的参数
在ts
中,一个类的参数如果带上修饰符,那个参数就变成了类的实例属性
class Mobile { constructor(readonly name: string = '小米') {} logName() { console.log(this.name); } }
上面的name
有修饰符,那么它就是Mobile
类的实例属性,等同于下面写法:
class Mobile { readonly name: string; constructor() { this.name = '小米'; } logName() { console.log(this.name); } }
创建服务
src/services/
下创建一个名叫comment
的服务:ng g s services/comment
// comment.service.ts import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class CommentService { constructor() { } }
- 被
@Injectable
装饰的类,就是一个可以被注入的类,也称为服务(为其它服务或者组件、指令、管道提供服务); -
元数据的 providedIn
配置表示是谁提供了这个服务,root
表示在根模块中提供。
使用服务
// src/data/comments.ts export default [{ "postId": 1, "id": 1, "name": "id labore", "email": "Eliseo@gardner.biz", "body": "laudantium enim quasi est quidem magnam voluptate ipsam" }, { "postId": 1, "id": 2, "name": "quo vero ", "email": "Jayne_Kuhic@sydney.com", "body": "est natus enim nihil est dolore omnis" }];
// comment.service.ts import { Injectable } from '@angular/core'; import comments from '../data/comments'; @Injectable({ providedIn: 'root' }) export class CommentService { constructor() { } getComments() { return comments; } }
创建一个service
组件获取服务:
// service.component.ts ... export class ServiceComponent implements OnInit { constructor(private commentService: CommentService) { console.log(this.commentService.getComments()); // 能够获取到模拟的数据 } // ... }
这就是使用依赖注入的便捷之处,如果没有,我们通过普通类的方法就该是这样了:
// comment.service.ts import { Injectable } from '@angular/core'; import comments from '../data/comments'; // 删除了@Injectable export class CommentService { constructor() { } getComments() { return comments; } }
... export class ServiceComponent implements OnInit { private comments = []; private commentService: CommentService; constructor() { // 这里就会使用 new 来实例化 this.commentServe = new CommentService(); console.log(this.commentServe.getComments()); } // ... }
提供服务的地方
一个服务的元数据默认配置是在根模块中提供,其实,它还可以由其他地方提供。
providedIn?: Type<any> | 'root' | 'platform' | 'any' | null
在服务本身的@Injectable()
装饰器中:
// xxx.service.ts @Injectable({ providedIn: 'platform' })
在NgModule
的@NgModule()
装饰器中:
@NgModule({ ... providers: [CommentService] })
在组件的@Component()
装饰器中:
@Component({ selector: 'app-service', templateUrl: './service.component.html', providers: [CommentService] })
服务,对于开发过程中处理一些公用的数据、方法是很有便捷的,就像开篇所说,可以大大提升开发效率和模块化程度。
本文转载自:公众号 岩弈 Angular教程,版权归原作者所有,本博客仅以学习目的的传播渠道,不作版权和内容观点阐述,转载时根据场景需要有所改动。
最新评论